Als een van de meer recente projecten heb ik een Memory game gemaakt in C# winforms.
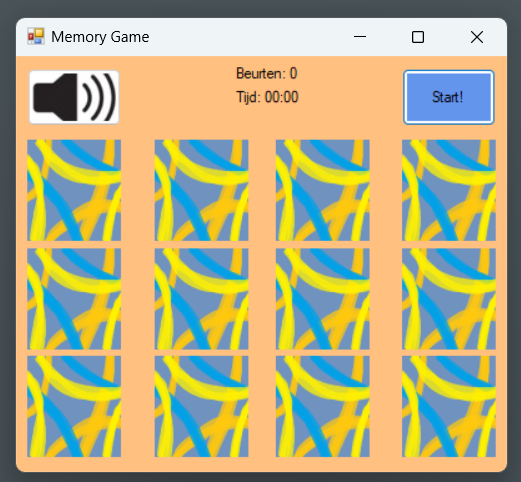
Ik heb voor dit project aardig wat regels code geschreven. Ik vond dit project redelijk makkelijk, maar een van mijn klasgenoten hielp mij met op gang komen, dus dat verklaart wat.
namespace MemoryGameProject
{
public partial class Form1 : Form
{
private PictureBox firstCard;
private PictureBox secondCard;
private bool canClick = false;
private int beurten = 0;
private DateTime startTime;
private int matchedPairs = 0;
private int totalPairs;
private bool soundOn = true;
public Form1()
{
InitializeComponent();
List<PictureBox> Thingamajigs = new List<PictureBox> { pictureBox1, pictureBox2, pictureBox3, pictureBox4, pictureBox5, pictureBox6, pictureBox7, pictureBox8, pictureBox9, pictureBox10, pictureBox11, pictureBox12 };
totalPairs = Thingamajigs.Count / 2;
Reset(Thingamajigs);
}
private void timerTime_Tick(object sender, EventArgs e)
{
DateTime currentTime = DateTime.Now;
TimeSpan elapsed = currentTime - startTime;
lblTijd.Invoke((MethodInvoker)delegate {
lblTijd.Text = "Tijd: " + elapsed.ToString(@"mm\:ss");
});
}
void Reset(List<PictureBox> Thingamajigs)
{
Random random = new Random();
List<string> tags = new List<string> { "appel", "appel", "bone", "bone", "boom", "boom", "eend", "eend", "melon", "melon", "memory", "memory" };
foreach (PictureBox Thing in Thingamajigs)
{
Thing.Image = Properties.Resources.backside;
int randomindex = random.Next(0, tags.Count);
Thing.Tag = tags[randomindex];
tags.RemoveAt(randomindex);
Thing.Click += Thingclick;
}
}
private void Thingclick(object sender, EventArgs e)
{
if (!canClick)
return;
if (soundOn == true)
using (SoundPlayer player = new SoundPlayer(Resources.CardTurn))
{
player.Play();
}
PictureBox DooDad = (PictureBox)sender;
string tag = DooDad.Tag as string;
System.Resources.ResourceManager rm = Properties.Resources.ResourceManager;
object Contraption = rm.GetObject(tag);
DooDad.Image = (System.Drawing.Bitmap)Contraption;
if (firstCard == null)
{
firstCard = DooDad;
}
else if (secondCard == null && DooDad != firstCard)
{
secondCard = DooDad;
canClick = false;
Timer timer = new Timer();
timer.Interval = 1000;
timer.Tick += new EventHandler(CompareCards);
timer.Start();
}
}
private void CompareCards(object sender, EventArgs e)
{
Timer timer = (Timer)sender;
timer.Stop();
beurten++;
lblBeurten.Text = "Beurten: " + beurten.ToString();
if (firstCard.Tag.Equals(secondCard.Tag))
{
if (soundOn == true)
using (SoundPlayer player = new SoundPlayer(Resources.CorrectJingle))
{
player.Play();
}
firstCard.Enabled = false;
secondCard.Enabled = false;
matchedPairs++;
if (matchedPairs == totalPairs)
{
if (soundOn == true)
using (SoundPlayer player = new SoundPlayer(Resources.VictoryJingle))
{
player.Play();
}
timerTime.Stop();
MessageBox.Show("Je hebt gewonnen!");
}
}
else
{
if (soundOn == true)
using (SoundPlayer player = new SoundPlayer(Resources.WrongJingle))
{
player.Play();
}
firstCard.Image = Properties.Resources.backside;
secondCard.Image = Properties.Resources.backside;
}
firstCard = null;
secondCard = null;
canClick = true;
}
private void btnStart_Click(object sender, EventArgs e)
{
canClick = true;
startTime = DateTime.Now;
timerTime.Enabled = true;
beurten = 0;
matchedPairs = 0;
lblBeurten.Text = "Beurten: 0";
lblTijd.Text = "Tijd: 00:00";
List<PictureBox> Thingamajigs = new List<PictureBox> { pictureBox1, pictureBox2, pictureBox3, pictureBox4, pictureBox5, pictureBox6, pictureBox7, pictureBox8, pictureBox9, pictureBox10, pictureBox11, pictureBox12 };
foreach (PictureBox Thing in Thingamajigs)
{
Thing.Image = Properties.Resources.backside;
Thing.Enabled = true;
}
Reset(Thingamajigs);
}
private void btnSound_Click(object sender, EventArgs e)
{
if (soundOn == true)
{
soundOn = false;
btnSound.Image = Properties.Resources.SoundOff;
}
else
{
soundOn = true;
btnSound.Image = Properties.Resources.SoundOn;
using (SoundPlayer player = new SoundPlayer(Resources.CorrectJingle))
{
player.Play();
}
}
}
}
}
Wat een leuk project